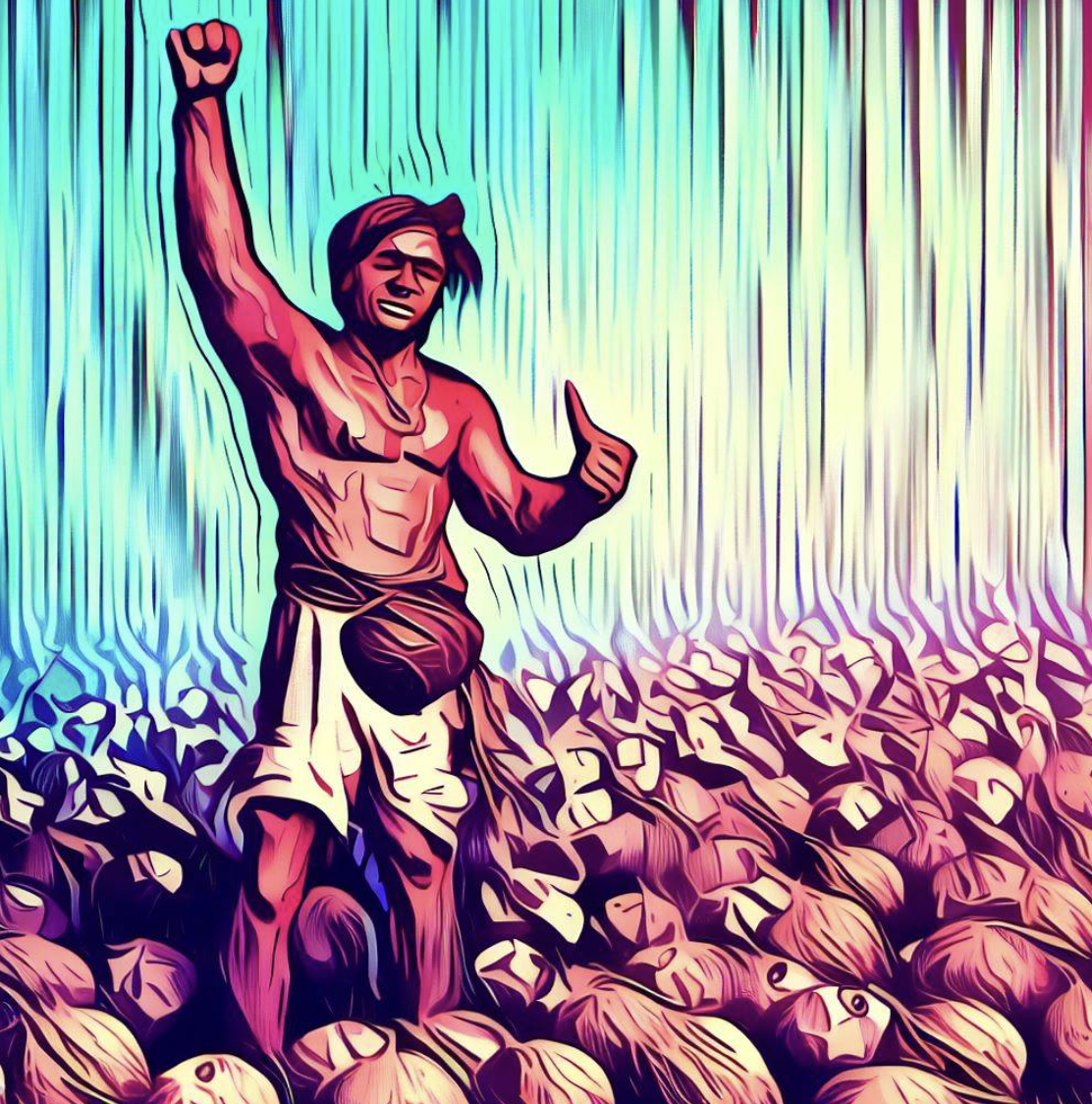
Counting the number of elements in sequences through compression
Contents
Counting the elements in a sequence is a common problem in mathematics, computer science, and programming. Although in many cases this appears tricky, there’s a simple method to make the task straightforward, requiring only basic math skills.
Examples Before Theory
Let’s dive into a few examples before explaining the method precisely.
Example 1: {5, 6, 7, …, 100}
First, find a function that maps integers (ideally starting from 0) to the elements above. In this case, it’s easy to see that $f(n) = 5 + n$. Now, we look for the value of $k$ that maps to 100. Using an equation, we find:
$$ f(k) = 100 \Rightarrow 5 + k = 100 \Rightarrow k = 95. $$
Thus, the sequence {5, 6, 7, …, 100} has 96 elements.
Example 2: {36, 39, 42, …, 141}
This example is trickier than before, but the same technique applies. The function for this sequence is $f(n) = 36 + 3n$. We want to find the $k$ that maps to 141:
$$ f(k) = 141 \Rightarrow 36 + 3k = 141 \Rightarrow k = 35 $$
The sequence {36, 39, 42, …, 141} has 36 elements.
Example 3: {87.3, 86.2, 85.1, …, -7.3}
This example demonstrates that the method works for any set of objects or numbers, as long as there’s a function to track the sequence elements and solvable equations for the function:
$$ f(n) = 87.3 - 1.1n $$
Find the edge case:
$$ f(k) = -7.3 \Rightarrow 87.3 - 1.1k = -7.3 \Rightarrow k = 86 $$
The sequence {87.3, 86.2, 85.1, …, -7.3} has 87 elements.
The Method Explained
Here’s a summary of the method:
- Identify the sequence $x_0, x_1, \cdots, x_k$.
- Find a function $f$ that describes the sequence. This function should be relatively easy to find based on the sequence behavior.
- Find the independent variables for which your function produces the two edge values.
- Calculate the result: $\text{final} - \text{start} + 1$
In step 4, finding an integer solution is required; otherwise, the function won’t reach the final number, and your function won’t truly track the sequence.
Although the examples above are linear, this method works for any bijection in the required range. Be cautious with more involved cases, as a non-bijective function can lead to double counting or missing numbers.
In essence, this approach condenses the sequence of interest into just two parameters: the function and the ending independent variable. The starting independent variable is also a parameter but can be inferred from the function.
For example, in the third case we have:
$$\{87.3, 86.2, 85.1, …, -7.3\} \equiv (f(n) = 87.3 - 1.1n, 86)$$
You can view this as a way of compressing the sequence.